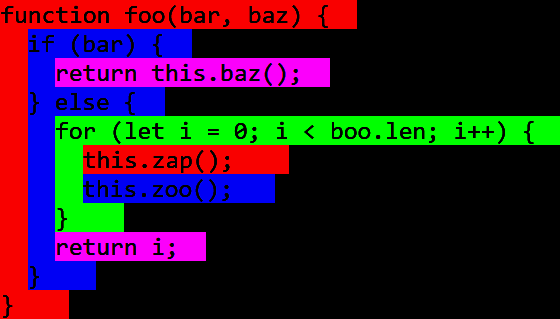
Basic Structure
The C code structure can be a bit intimidating for some at the first try, but it’s very simple and becomes intuitive over time!
In C, programs are made up of statements. Statements end with a semicolon and are organized into sections called functions.
Let’s go back to the code example we used earlier:
#include <stdio.h>
int main() {
printf("SNAKE Security\n");
return 0;
}
You could write this code like this:
#include <stdio.h>
int main() {printf("SNAKE Security\n");return 0;}
As long as the statements conform to the standards, the compiler doesn’t care how they are written.
Although this code works, it’s ugly and chaotic… That’s why we have the proper structure and style of code to use.
K&R
There is a lot of debate about this, but although some “programmers” code in other ways, there is only one official style standard and that is K&R, which was documented in the book The C Programming Language written by Brian Kernighan and Dennis Ritchie (the creator of C).
The table below is a list of the styles in use…
Name | Example | Open/close equal height |
Open/close equal indent |
Open/close don’t share lines with content |
---|---|---|---|---|
K&R | ![]() |
✓ | ✓ | ✓ |
Allman | ![]() |
𐄂 | ✓ | ✓ |
GNU | ![]() |
𐄂 | 𐄂 | ✓ |
Whitesmiths | ![]() |
𐄂 | 𐄂 | ✓ |
Horstmann | ![]() |
𐄂 | ✓ | 𐄂 |
Pico | ![]() |
𐄂 | 𐄂 | 𐄂 |
Ratliff | ![]() |
✓ | 𐄂 | ✓ |
Lisp | ![]() |
✓ | 𐄂 | 𐄂 |
For me, anything other than K&R is nothing but disgusting…
In K&R, to reflect their equal importance in terms of impact on the meaning of the code above and below them, the space that the opener and closer of a control structure occupy on the screen is equal (one line).
K&R style code does not have any artificial, meaningless lines as a result of style enforcement. Each line tells the reader something they wouldn’t otherwise know, and they can therefore move from line to line, picking up new information at each one.
Blank lines are not bad, since they are useful for grouping related lines together and separating unrelated lines, and so hitting a blank line is a sign, like a paragraph or section break in a book, that the old topic ends and a new topic begins, but whether a blank line is appropriate in a given context is entirely up to that context, and so cannot be decided by the coding style.
In K&R, each empty line has a purpose, determined by the programmer based on the context, to communicate some information about the relationship of each line.
Indentation
Look, C is not a dumb language like Python, so there is no convention or requirement to indent code.
Although it is good practice to follow the indentation style of the K&R specification…
Comments
The ability to comment code is extremely useful in complex programs… it’s good practice to make comments either for yourself in the future or for other programmers…
For simple “side notes” that explain what certain parts of the code do, single-line comments are particularly useful. The best locations for these comments are after variable declarations, and after parts of the code that might need explaining.
// Example of a single-line comment
For long explanations of code, multi-line comments are most useful. They can be used for copyright/license notices, and they can also be used for explanations of the purpose of a block of code or functions.
/* Example of multi-line comment
function : int main()
return : 0 at end
procedure : prints "SNAKE Security" with a new line
*/
/**********************************************
* This is also a multi-line comment *
* But done with a cool, eye-catching style *
* *
* This is how we do! *
**********************************************/