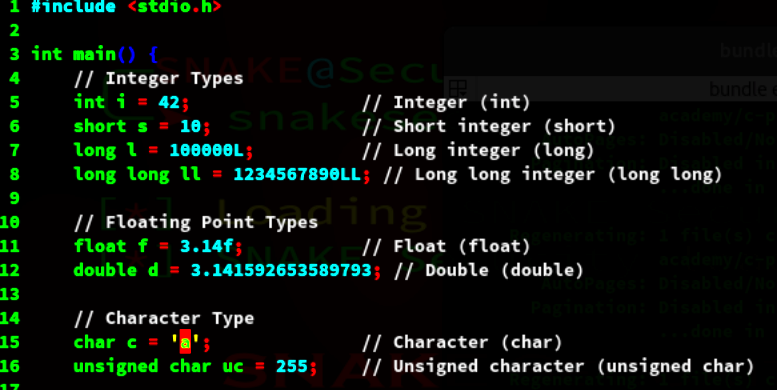
Variables
In simple terms, a variable is a human-readable word or name that stores or points to a value in memory…
It’s a very important part of any programming language, so let’s take some time to understand how they work in C.
Before using a variable, you must declare it, using the following syntax:
data_type variable_name;
-
data_type : In this context “data_type” refers to the type of the variable, each variable has a type to which it belongs… this determines the type of data the variable can store…
-
variable_name : This is the actual name of the variable, it’s how you’ll call it in your code.
To assign a value to a variable, you can simply use the following syntax:
variable_name = value;
- value : This obviously refers to the value you want to store in this variable
Primitive Data Types
Primitive data types are the most basic data types used to represent simple values such as integers, floats, characters, etc.
int
int is used to store integer numbers (any number, including positive, negative, and zero, without a decimal part). Octal values, hexadecimal values, and decimal values can be stored in the int datatype in C.
- Range : -2,147,483,648 to 2,147,483,647
- Size : 4 bytes
- Format Specifier : %d
Example:
int age; // Declares the variable "age" of type "int
age = 27; // Assigns the value 27 to the "age" variable
char
The char data type allows only a single character to be stored in its variable. The size of the character is 1 byte in size. It is the simplest datatype of C. It stores a single character and requires a single byte of memory.
- Range : -128 to 127 or 0 to 255
- Size : 1 byte
- Format Specifier : %c
Example:
char letter_a;
letter_a = 'a';
float
In C programming, the float data type is used to store floating-point values. Float stores decimal and exponential values in C. It is used for single-precision storage of decimal numbers (numbers with floating-point values).
- Range: 1.2E-38 to 3.4E+38
- Size: 4 bytes
- Format Specifier: %f
Example:
float cash_in_bank;
cash_in_bank = 12.75;
double
A double data type in C is used to store decimal numbers (numbers with floating point values) with double precision.
The double data type is basically a precision type that can hold 64-bit decimal numbers or floating point numbers. Since double has more precision as compared to the float then it is much more obvious that it occupies twice the memory that the floating-point type occupies. It can easily hold about 16 to 17 digits before or after a decimal point.
- Range: 1.7E-308 to 1.7E+308
- Size: 8 bytes
- Format Specifier: %lf
Example:
double big_double_number;
big_double_number = 123456789.123456;
void
The void data type in C is used to indicate that no value exists. It does not return a result value to its caller. It has no values and no operations. It is used to represent nothing. Void is used in a variety of ways: as the return type of functions, as void function arguments, and as pointers to void.
Data type modifiers
In C, the size and/or range of a basic data type can be adjusted using datatype modifiers.
-
signed: This modifier allows a type to store both negative and positive numbers. By default, most types are signed (so “int” is “signed int”).
-
unsigned: This one restricts the type to only positive values and zero. It gives you more positive range at the cost of not being able to store negative numbers.
signed int a = -10; // can hold negative and positive values
unsigned int b = 10; // only positive values
-
short: This modifier reduces the size of a type (usually half the size of the standard one). It’s typically used with “int”.
-
long: This one increases the size of a type (usually double the size of the standard one).
-
long long: This is even longer—often providing a size twice that of “long”.
short int small = 32767; // smaller range
long int large = 123456789; // larger range
long long int very_large = 1234567890123456789; // huge range
- long long with unsigned : You can combine “long long” with “unsigned” for some *seriously big numbers.
unsigned long long int big_unsigned = 18446744073709551615;
The following table will help you better understand the modifiers:
Modifier | Size (in bytes) | Range (32-bit) | Format Specifier |
---|---|---|---|
signed int | 4 | -2,147,483,648 to 2,147,483,647 | %d |
unsigned int | 4 | 0 to 4,294,967,295 | %u |
short int | 2 | -32,768 to 32,767 | %hd |
unsigned short int | 2 | 0 to 65,535 | %hu |
long int | 4 | -2,147,483,648 to 2,147,483,647 | %ld |
unsigned long int | 4 | 0 to 4,294,967,295 | %lu |
long long int | 8 | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 | %lld |
unsigned long long | 8 | 0 to 18,446,744,073,709,551,615 | %llu |
signed char | 1 | -128 to 127 | %c |
unsigned char | 1 | 0 to 255 | %hhu |
-
Use “unsigned” when you know your values can never be negative and you want to maximize the range of positive numbers.
-
“short” and “long” are good for adjusting memory usage or working with very large numbers when precision is important.
-
Use “long long” if you need to store exceptionally large numbers, like for handling big data.
Here is a small code example that illustrates what we’ve learned so far:
#include <stdio.h>
int main() {
// Integer Types
int i = 42; // Integer (int)
short s = 10; // Short integer (short)
long l = 100000L; // Long integer (long)
long long ll = 1234567890LL; // Long long integer (long long)
// Floating Point Types
float f = 3.14f; // Float (float)
double d = 3.141592653589793; // Double (double)
// Character Type
char c = 'a'; // Character (char)
unsigned char uc = 255; // Unsigned character (unsigned char)
printf("Integer (int): %d\n", i);
printf("Short integer (short): %d\n", s);
printf("Long integer (long): %ld\n", l);
printf("Long long integer (long long): %lld\n", ll);
printf("Float (float): %f\n", f);
printf("Double (double): %f\n", d);
printf("Character (char): %c\n", c);
printf("Unsigned char (unsigned char): %hhu\n", uc);
return 0;
}
Derived Data Types
Derived Data Types are derived from Primitive Data Types!
C provides three derived data types:
- Function
- Array
- Pointer
User-Defined Data Types
User-Defined Data Types are those defined by the user. These data types are derivatives of the existing data types.
There are 4 types of user-defined data types in C. They are:
- Structure
- Union
- Enum
- Typedef
Now things get a bit more complicated and you need to pay close attention, so I won’t go into that in this lesson, but rather do separate lessons for better understanding.
Don’t worry, you’re going to understand it all in the next few lessons…