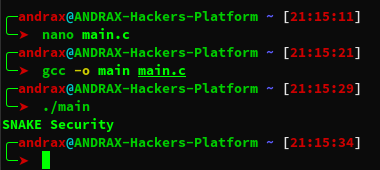
C Programming Language Intro
Introduction to C The Language of Gods, History, What is C and how to program it...
Disclaimer
This content is provided for educational and informational purposes only. The techniques and tools discussed, are intended to raise awareness about security risks and help developers and system administrators protect their systems.
We do not encourage, support, or condone any form of unauthorized access, exploitation, or malicious activity. All demonstrations were conducted in controlled environments with proper authorization.
Use this knowledge responsibly and always adhere to your local laws and ethical guidelines. Hacking should only be performed in environments where you have explicit permission.
The author assumes no responsibility for any damages or legal consequences arising from the misuse of this content. Always ensure you have proper authorization before testing or auditing any system!
C History
Those who do not learn history are doomed to repeat it. – George Santayana.
Dennis Ritchie and Ken Thompson developed the C programming language at Bell Telephone Laboratories in 1972.
Brian Kernighan was co-author with Dennis Ritchie of The C Programming Language (K&R 1988).
In 1983, the American National Standards Institute (ANSI) formed the X3J11 committee to create a standard C specification, and in 1989 the C standard was ratified as ANSI X3.159-1989, “Programming Language C”. This 1989 language version is called ANSI C or C89.
The complete history of C on wikipedia
Until now, the C language standards are:
Year | Informal name | Official standard |
---|---|---|
1972 | first release | — |
1978 | K&R C | — |
1989, 1990 | ANSI C, C89, ISO C, C90 | ANSI X3.159-1989, ISO/IEC 9899:1990 |
1999 | C99, C9X | ISO/IEC 9899:1999 |
2011 | C11, C1X | ISO/IEC 9899:2011 |
2018 | C17, C18 | ISO/IEC 9899:2018 |
2024 | C23, C2X | ISO/IEC 9899:2024 |
Future | C2Y | — |
C is so well structured and developed that it does not need to be updated every week like other languages; each standard implements new features as guidelines.
What is C
Even though it was first developed in 1970, C is still a very popular programming language and is used in practically every piece of software we use today!
While some “modern” languages may include new features, their compilers and interpreters are written in C… in the end, everything used in programming is just a C wrapper.
If you wish to make an apple pie from scratch, you must first invent the universe. - Carl Sagan
C is a general-purpose programming language, meaning that anything can be implemented.
But it will make you work for it!
Nowadays, some idiots people say that C is a low-level language, which is not true because C was designed to be high-level, but most of those who call themselves programmers today don’t really know how to program, they just connect api with some syntax.
Every new programming language we have today, Python, GO, Ruby, PHP… are nothing more than wrappers of C, the compilers and/or interpreters of these languages are in C so I reaffirm that what you call programming languages do not exist, they are basically APIs because in the end what is really being executed is C.
Before you talk about C++, it’s nothing more than C with a few additions and, in my opinion, a lot of descriptive stuff… I like to think of C++ as “C made for women”.
C is portable to all existing hardware, every platform has a C compiler, GCC (GNU C Compiler), which we’ll talk about later, covers almost every architecture.
In cybersecurity, we use C for advanced hacking, the development of exploits, the creation of tools and protocols, and much more.
How to program in C
The C programming environment should already be installed if you’re a Linux user. If not, install GCC, which is the compiler we’ll be using from now on… IDE or text editor, it’s up to you…
Note that if you use ANDRAX-NG, everything is already ready for you to program…
Since everything should be ready, let’s start with our first C program:
#include <stdio.h>
int main() {
printf("SNAKE Security\n");
return 0;
}
We’ll compile using GCC
gcc -o main main.c
Note that we pass the flag (-o) with the “main” parameter and then pass “main.c”…
- -o : Specifies the name of the file that will result from the compilation, i.e. the name of the program itself…
- main : The name we chose for the program
- main.c : The source file or files that we are going to compile, in our case just a single .c file
Now just run the program, the result of the compilation and execution is shown below
Okay… we’ve made a program that displays a message in the terminal, but what does each line of this program actually do?
Hold on to your butt because I’m going to explain…
#include <stdio.h>
“#include” It tells the C preprocessor to grab the contents of some other file and insert it right there in the code.
In our case, we’ll be inserting the stdio.h (.h is for header) library, which is the C “Standard Input/Output” library.
Anything that starts with a hash/hashtag or pound sign (#) is something that the preprocessor works on before the compiler even starts. Common preprocessor directives, as they’re called, are #include and #define. More about this later.
int main() {...}
Now things are getting more interesting, but also more complex, so I’ll just explain the basics, because you’ll understand everything later on…
In this line we declare our initial function, which is always called “main” in C, because the compiler needs to know where to start…
The “int” is to specify what our function returns, which in our case is a value of type int, it could be of type “void” if it didn’t return anything…
The parentheses ”( )” are parameters of the function in this case we don’t use any
Everything inside the brackets ”{ }” is part of the function
printf("SNAKE Security\n");
This is the magic line that makes our message appear…
Here we are invoking the “printf” function from the “stdio.h” library and passing the message “SNAKE Security\n” as a parameter, the “\n” character is the “newline” that tells the operating system the line ends there.
Semicolons ”;” are end statements in C. It indicates that the current statement has ended, and other statements that follow are new statements.
return 0;
This line defines the return code of the function since the “main” function is being started as an int function… as it is the only function in this program, this value will be passed to the operating system on exit… if we change the value from 0 to 1, the operating system will understand it as an error code because any return above 0 is an error… we’ll talk more about this later…